Magical Girl Saga Devlog[3]
Implementing the Dialogue And Day/Night Cycle Systems
Hello everyone and welcome to devlog #3 for my 2D JRPG indie game, Magical Girl Saga! In this devlog, I'll be discussing how I implemented the dialogue system for Mahou Shoujo Monogatari and going through the design and thought process behind why I chose what I chose for the dialogue system. Without further ado, let's dive right into today's devlog!
Part I - Dialogue System
Displaying The Dialogue
The first step in displaying the dialogue was to figure out how I wanted to display dialogue. I knew I wanted the currently speaking character to have their portrait in a frame next to the dialogue box and I also wanted to implement character by character text scrolling with a sound effect to make it sound like the characters are talking. So the first thing I did was design a prototype dialogue window for the dialogue and character portrait to be displayed and I came up with this:
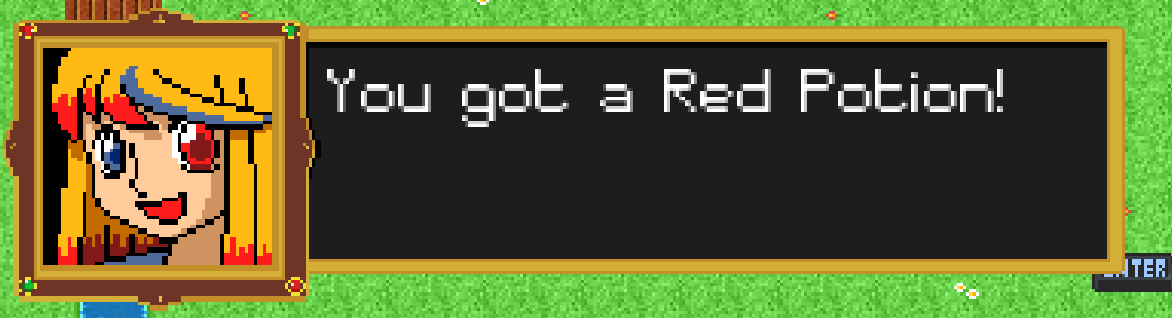
After getting the prototype finished and implemented into the game, it was time to figure out how the dialogue should be stored. I opted to use a 2D array, as this will allow for a dialogue set and a dialogue index, allowing for different dialogues to play for the same object or character when interacted with.
Implementing 2D Arrays
So my first thought was to use a 2D array with size 20 on both dimensions, so I created a new class, Entity, that objects and characters can extend from and created a simple method that populates the array with null values so we can then override those null values within the object and character classes to set the dialogue and I did this using the following code:
using Godot;
using System;
public partial class Entity : Node
{
public string[,] dialogues = new string[20,20];
public int dialogueIndex = 0;
public int dialogueSet = 0;
public override void _Ready() {
for (int i = 0; i < 20; i++) {
for (int j=0; j < 20; j++) {
dialogues[i, j]=null;
}
}
}
}
Now that the initialisation of the dialogues array is set, we can now successfully store dialogue as sets and have multi-line dialogue in my game. Now the next step was to "animate" the text by having it scroll across the screen character by character.
Scrolling Text
So the next thing I did was to programmatically make the text display character by character for each line of dialogue. I did this by using a Timer and by splitting the dialogue by line and iterating through it to put the characters in a char array for iteration through that new char array to them use the timer to display the characters one by one at a time. I then implemented a sound effect to play every time a character is displayed on the screen, which creates a sort of talking effect.
//Within The drawDialogue method
dialogueBox.Visible = true;
ui.Visible = false;
foreach (string line in currentDialogue.Split("\n"))
{
dialogueText.Text = line;
}
characters = currentDialogue.ToCharArray();
for (charIndex = 0; charIndex < characters.Length; charIndex++) {
timer.Start(0.025); combinedText
+=characters[charIndex];
currentDialogue=combinedText;
dialogueText.Text=combinedText;
}
private void _on_timer_timeout()
{
dialogueText.VisibleCharacters++;
if (talkSound != null)
{
audioPlayer.Stream = talkSound;
audioPlayer.Play();
}
if (dialogueText.VisibleCharacters >= characters.Length)
{
timer.Stop();
dialogueText.VisibleCharacters = -1;
audioPlayer.Stop();
}
}
After this, the dialogue system is now implemented into Magical Girl Saga! Now it's time to move onto the Day/Night cycle.
Part II - Day/Night Cycle
Day/Night Cycle System Using CanvasModulate
In Godot 4, there's a unique node known as the CanvasModulate, which applies a tint to the game window while also allowing things like point lights and dynamic lighting to be visible through the tint. I wanted to use CanvasModulate because it allowed for the darkness effect I was going for in my game while also allowing dynamic lighting, so I went to work on getting CanvasModulate to work and thankfully, while it did take me a while to figure out, the solution was quite simple: all I had to do was use the AnimationPlayer node to create two animations, one for switching from day to night and another for switching from night to day. Once the two animations were implemented, I was then able to program day states to allow for a set amount of time for it to be day or night while also playing the animations when applicable and I came up with this solution:
using Godot;
using System;
public partial class DayNightCycle : CanvasModulate
{
public int dayState = 0; // 0 for day, 1 for night
public int dayLengthInTicks = 600000; // Equal to 10 minutes in-game time
public int dayTimeTicks = 0;
PointLight2D pointLight;
AnimationPlayer aniPlayer;
public override void _Ready()
{
pointLight = GetNode("/root/GameLevel/PlayerJeanne/pointLight");
aniPlayer = GetNode("/root/GameLevel/DayNightCycle/AnimationPlayer");
// Initialize the tick counter and day state
dayTimeTicks = 0;
dayState = 0;
pointLight.Visible = false;
aniPlayer.Play("nightToDay");
}
public override void _Process(double delta)
{
// Increment the tick counter
dayTimeTicks++;
// Check if it's time to switch states
if (dayTimeTicks >= dayLengthInTicks)
{
dayTimeTicks = 0; // Reset the tick counter
// Switch day/night state
if (dayState == 0)
{
dayState = 1;
changeToNight();
pointLight.Visible = true;
}
else
{
dayState = 0;
changeToDay();
pointLight.Visible = false;
}
}
}
public void changeToDay()
{
aniPlayer.Play("nightToDay");
}
public void changeToNight()
{
aniPlayer.Play("dayToNight");
}
}
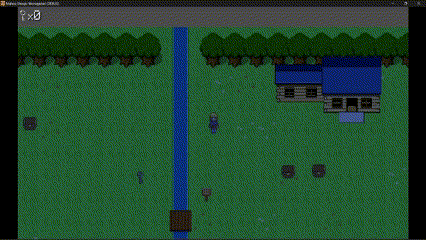
Conclusion
Well, that's all the progress I made this time on Magical Girl Saga! Next is a return to the inventory system and hopefully the start of NPCs. Until next time, Adieu!